Do I need to enable debug data on the physics render to view a single raycast?
Debug RayCast Ray
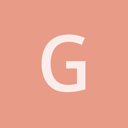
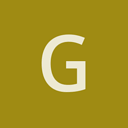
You can use DebugRenderer to draw line… There are many posts on this…
e.g.
DebugRenderer* debug = scene_->GetComponent();
debug->AddLine(Vector3(0, 0, 0), Vector3(mx, my, 0), Color(1, 1, 1, 1), false);
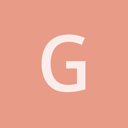
Oddly I tried this before creating this thread. For some reason it will not render the line. I use it for other things and it works fine.
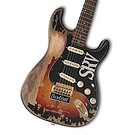
You need to draw things after the scene drawing is finished. Subscribe to the E_POSTRENDERUPDATE and do your drawings there.
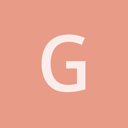
Okay I will try this. Seems odd though. I don’t do this for other cases and the lines render fine.
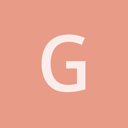
So I subscribe to the E_POSTRENDERUPDATE. I can see the ray briefly before it disappears. I’m try to raycast forward from a rocket. The line seems to be aiming right of the rocket projectile not it’s forward facing. Maybe I have a mistake in my raycast.
PhysicsWorld* physicsWorld_ = scene_->GetComponent<PhysicsWorld>();
PhysicsRaycastResult result;
Vector3 pos(boxNode->GetWorldPosition());
Ray ray(pos, boxNode->GetWorldDirection().FORWARD); // the given vector is the direction
physicsWorld_->RaycastSingle(result, ray, 250.0f, 1);
debug->AddLine(pos, result.position_, Color::YELLOW,false);
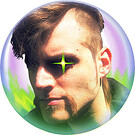
Remove the
.FORWARD
: A
Node
's direction is already the local forward. The
constant
Vector3::FORWARD holds a
{ 0.0f, 0.0f, 1.0f }
vector. Similarly a
Node
has
GetUp()
and
GetRight()
functions which return
rotation_ * Vector3::UP
and
rotation_ * Vector3::RIGHT
respectively.
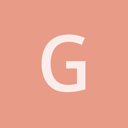
I removed the FORWARD part. The ray is still off. It does not travel the same direction as the moving rocket projectile.
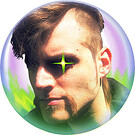
Does it use physics? If so, you may want to use
rigidBody_->GetLinearVelocity().Normalized()
instead of the
Node
's direction.
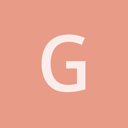
Okay I will try this
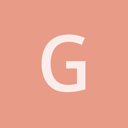
So I got the rigid body and used the
GetLinearVelocity().Normalized()
, but the same thing still happens.
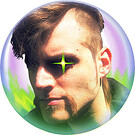
Could you share a screenshot or video?
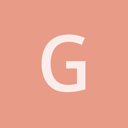
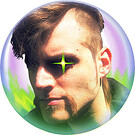
The line
does
seem aligned with the launcher. Maybe a
DebugRenderer::AddNode(boxNode)
could help to understand what is going on. Did you check the
asset
’s alignment?
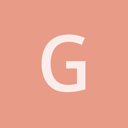
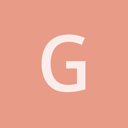
boxNode->SetPosition(Pos + Vector3(0.0f,0.0f,1.0f));
boxNode->SetRotation(objectNode->GetWorldRotation() * Quaternion(-90, Vector3(0, 1, 0)));
Pos is the launchers world position. I move it forward slightly.
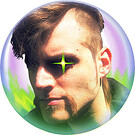
If your asset is not oriented correctly, modify the asset . When this is no option, the correction should happen by attaching the model component to a child node and rotating that (90 degrees left) as to not mess up the logic with it.
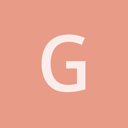
Okay I will give this a try.
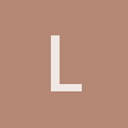
Is the line pointing towards the world position 0,0,0? You should check if there is a hit, because if not result.position_ will be Vector3::ZERO
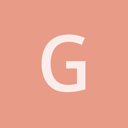
That sounds about right. The ray always tries to point a certain direction no matter how much the character rotates.
Added this.
if (result.distance_ < 250)
{
objectNode->SetWorldPosition(result.position_);
}
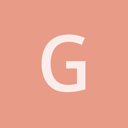
So after more testing the ray is always to the left of the rocket. Even if I turn the character in the opposite direction.
Rocket in 3d max I don’t see any issues:
EDIT: I disabled the physics on the rocket and rotated it in 3ds max and rest it’s transofrmation. The rocket and the ray cast now point in the right direction. I just need to track down what the issue is.
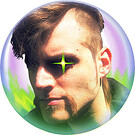
See how the rocket points in the positive direction of the X-axis? Foward in 3DsMAX is the direction the green Y arrow points to, which in Urho will be positive Z and correlates to the direction of the ray.
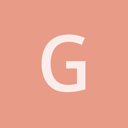
I rotated it to positive Y axis. Does this mean for every halo model I will have to reorient the models?
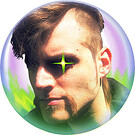
That would seem likely.
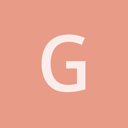
Also I found another thing that was causing the issue. In my projectile class I had this line:
body->SetLinearVelocity(parentnode_->GetWorldRotation() * Vector3(0.0f, 0.0f, 1.0f) * speed_);
This cause the rocket to rotate wrong. How can I get the rocket to spawn slightly in front of the launcher, but use the character or launchers forward that way the rocker and ray are correct and not lopsided.
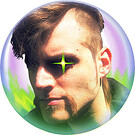
Instead of setting the velocity of a rigid body directly, one should prefer applying forces and impulses.
You can use
Newton’s 2nd law
to convert one to the other:
force = mass * acceleration
ApplyImpulse
is expected to be used as a single-shot push, wheras
ApplyForce
expects the force to be multiplied by the timestep of the
FixedUpdate
event.
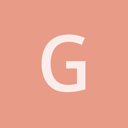
@Modanung ApplyImpulse works great. Only down side is I cannot get the rocket to travel in the forward direction of the character. If I rotate the character and fire the rocket. The rocket travels the same.
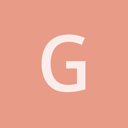
Okay I got it working correctly now.
CODE:
boxNode->SetRotation(objectNode->GetRotation());
This sets the projectile node to the characters rotation. Then the physics with ApplyImpulse moves it in the forward facing direction. The ray cast is also spot on now.
Thanks @Modanung
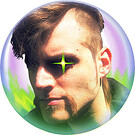
Do note that
SetRotation
and
GetRotation
are in
local transform space
, meaning this line will only function fine when the parents of both nodes have identical world space transforms. It will fail - for instance - if Master Chief would launch a missile while riding a bicycle.
To be safe, you can use
SetWorldRotation
and
GetWorldRotation
instead.
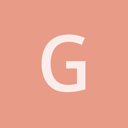
Okay I will update that. Thanks man